I am having troubles using the try catch statement. I am using this statement to avoid the code to stop when an image is not found because I would like to go on with the next scripts. However, using the following code, Eggplant stops on the not found image (“ETM_review/Action”) and opens the window to propose a solution. I have to click on “proceed” to continue the execution of the code. I was expecting the error to be catched in the “catch” section. Am I missing something ?
Try
Click "ETM_review/Action"
Catch
LogError "An error occured while executing the script" & my name
End Try
You have to add in error handling logic for the click
Try
if ImageFound(ImageName: “/path/to/image/”, waitFor: x seconds)
Click “ETM_review/Action”
else
throw “Image not found”
Catch
LogWarning “Use a log warning instead of a log error. This way your script pushes forward and you can analyze the logs later. You may want to accomplish more in the script and not want it to fail out right away”
end try
Also, check your image and image path. If your image is not cropped properly, Eggplant will not find it. Also, if the path is not correct, that may be the issue as well. This may be alleviated with search rectangles or with proper cropping. When cropping, make sure that there are no pixels in the image that can throw Eggplant off.
Another issue can be timing. That is what the “waitFor” is for.
Thanks Stephen, that reply is exemplary and very helpful because our team often come to our lead developer with exactly this issue. The nub of the problem is often that they haven’t handled the resulting event quite right.
Hi Stephen,
Thank you for your answer. So there’s no other way to ignore an image not found than using an “if/else” and throw an exception ? I am testing a web application (IBM ELM suite) and I created a lot of script that are doing a unique action (like creating a test case). These tests will be run during the night mostly, so I would like to avoid an error to stop the execution of the scripts (it can be Image not found or anything else). If one script cannot be run, I would to continue with the next one.
How can I do this without using an if/else structure at every line of my script ?
Dorian
Okay I found the solution : I just needed to go to “Run” tab, “Image update” and check “Update off (throw exception)”
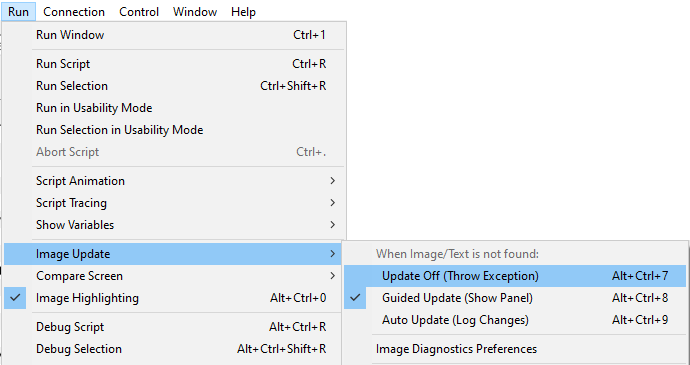
You don’t have to do a bunch of if/else statements. I was just giving an example of a solution. I like to know whether or not something was found and so I implement error handling to make sure. Also, if you want your script to continue without bailing out, use a LogWarning.
You can also pass a parameter to the function called “ETM” and if the parameter is equal to “ETM”, that image is looked for and clicked. Else if the image is not clicked, throw a warning.
// create search rectangle for whatever buttons
put [[X1, Y1]], [[X2, Y2]] into SR
set SearchRectangle to SR
to FunctionName
set etm_parameter = “ETM”
set another_parameter = “another_random_thing_you_are_testing”
set another_parameter_2 = “another_random_thing_you_are_testing_2”
if etm_parameter is equal to “ETM” then
// will look for image and wait up to 30 seconds to find it - if found before 30 sec, script will move on
if ImageFound(ImageName: “/path/to/image/”&etm_parameter, waitFor:30) then
Click “/path/to/image/”&etm_parameter
else
LogWarning “ETM Review button NOT found! - Please analyze logs for troubleshooting!”
end if
set SR to empty
end FunctionName
Also, don’t underestimate the power of search rectangles based on coordinates vs. images. Sometimes search rectangles based on images are your best friend, especially if what you are looking for is not static. If what you are looking for never changes positions on the GUI, then use coordinates.
Hi Stephen
I’m not sure I understand the example script you provided. Is ETM a built-in function or is this standing for an image that you’re searching for called ETM and why are another_parameter and another_parameter_2 in the example set but not referenced in the function called FunctionName please?
Thanks
It is a just a general example of code that needs to be tailored to what you need. ETM came from the original question.